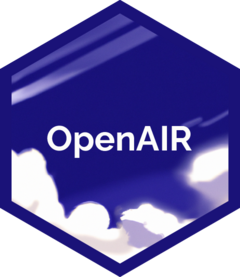
Check if a text file or character string contains an R function definition
contains_r_func.Rd
This function parses a text file or a character string and returns TRUE if it contains a valid R function definition. It uses a regex pattern to identify possible R function definitions and then attempts to parse the matched lines as R code.
Arguments
- input
A character string specifying the path to the text file, or a character string containing the text to be checked for an R function definition.
Value
A logical value (TRUE or FALSE) indicating whether the input contains a valid R function definition.
Examples
# Create a temporary file with an R function definition
temp_file <- tempfile(fileext = ".R")
writeLines("example_function <- function(x) {\n return(x * 2)\n}",
temp_file)
# Check if the temporary file contains an R function definition
result <- contains_r_func(temp_file)
print(result) # Should print TRUE
#> [1] TRUE
# Check if a character string contains an R function definition
result <- contains_r_func(
"example_function <- function(x) { return(x * 2) }")
print(result) # Should print TRUE
#> [1] TRUE
# Remove the temporary file
file.remove(temp_file)
#> [1] TRUE