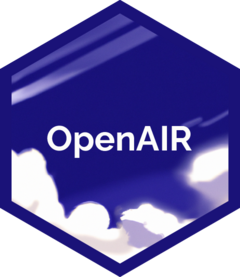
Generate Text Using the OpenAI API's Chat Endpoint
chat_completion.Rd
This function generates natural language text in a conversational style using the OpenAI API's chat endpoint. It takes a series of chat messages as input, either as a data.frame or a chatlog object, and generates a text completion based on the conversation history and the specified model parameters.
Usage
chat_completion(
msgs,
functions = NULL,
function_call = NULL,
model = "gpt-3.5-turbo",
temperature = NULL,
max_tokens = NULL,
n = NULL,
stop = NULL,
presence_penalty = NULL,
frequency_penalty = NULL,
best_of = NULL,
logit_bias = NULL,
stream = FALSE,
top_p = NULL,
user = NULL
)
Arguments
- msgs
A data.frame containing the chat history to generate text from or a chatlog object
- functions
An optional list of functions to use for the function call.
- function_call
An optional list specifying the function call to use.
- model
A character string specifying the ID of the model to use. The default value is "gpt-3.5-turbo".
- temperature
An optional numeric scalar specifying the sampling temperature to use.
- max_tokens
An optional integer scalar specifying the maximum number of tokens to generate in the text.
- n
An optional integer scalar specifying the number of text completions to generate.
- stop
An optional character string or character vector specifying one or more stop sequences to use when generating the text.
- presence_penalty
An optional numeric scalar specifying the presence penalty to use when generating the text. The default value is NULL.
- frequency_penalty
An optional numeric scalar specifying the frequency penalty to use when generating the text. The default value is NULL.
- best_of
An optional integer scalar specifying the number of completions to generate and return the best one. The default value is NULL.
- logit_bias
An optional named numeric vector specifying the logit bias to use for each token in the generated text.
- stream
An optional logical scalar specifying whether to use the streaming API. The default value is
FALSE
.- top_p
An optional numeric scalar specifying the top p sampling ratio. The default value is NULL.
- user
A unique identifier representing your end-user, which can help OpenAI to monitor and detect abuse.
See also
https://platform.openai.com/docs/ for more information on the OpenAI API.
Examples
if (FALSE) {
openai_api_key("your_api_key_here")
msgs_df <- data.frame(
role = c(
"system",
"user",
"assistant",
"user"
),
content = c(
"You are a helpful assistant",
"Who won the world series in 2020?",
"The Los Angeles Dodgers won the World Series in 2020.",
"Where was it played?"
)
)
chat_completion(msgs_df)
}